With the advent of advanced hardware and software technologies, deep learning algorithms can now process and analyze vast amounts of data, making it possible to extract insights and patterns that were once impossible to uncover.
One of the most popular deep learning libraries is Tensorflow, an open-source software library developed by Google. Tensorflow provides a flexible framework for building and training deep neural networks, and supports a wide range of platforms and devices. In this blog post, we will explore the basics of Tensorflow and deep learning with code examples.
Getting Started with Tensorflow
To get started with Tensorflow, you first need to install the library on your computer. You can install it using pip, which is the Python package installer. Here’s the command to install Tensorflow using pip:
pip install tensorflow
Building a Neural Network with Tensorflow
Neural networks are the building blocks of deep learning algorithms. They are designed to simulate the behavior of the human brain, allowing machines to learn from data and make predictions. Tensorflow provides a flexible framework for building neural networks, allowing you to customize the architecture of your model to suit your specific needs.
Here’s an example of how to build a neural network using Tensorflow:
import tensorflow as tf
# Create a Sequential model
model = tf.keras.models.Sequential()
# Add a dense layer with 64 units and ReLU activation
model.add(tf.keras.layers.Dense(64, activation='relu', input_shape=(10,)))
# Add another dense layer with 64 units and ReLU activation
model.add(tf.keras.layers.Dense(64, activation='relu'))
# Add an output layer with 1 unit and sigmoid activation
model.add(tf.keras.layers.Dense(1, activation='sigmoid'))
# Compile the model with binary crossentropy loss and Adam optimizer
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
In this example, we create a Sequential model, which is a linear stack of layers. We add a dense layer with 64 units and ReLU activation, followed by another dense layer with 64 units and ReLU activation. We then add an output layer with 1 unit and sigmoid activation. Finally, we compile the model with binary crossentropy loss and Adam optimizer.
Training the Neural Network
Now that we have built our neural network, we can train it on a dataset of examples. For this example, we will use the Pima Indians Diabetes Dataset, which contains information about the medical history of Pima Indians and whether or not they developed diabetes. We will use 75% of the data for training and 25% for testing.
# Load the Pima Indians Diabetes Dataset
dataset = tf.keras.datasets.pima_indians_diabetes
# Split the data into training and testing sets
(x_train, y_train), (x_test, y_test) = dataset.load_data(test_split=0.25)
# Normalize the input data
x_train = x_train / 255.0
x_test = x_test / 255.0
# Train the model for 100 epochs
model.fit(x_train, y_train, epochs=100, validation_data=(x_test, y_test))
In this example, we load the Pima Indians Diabetes Dataset and split the data into training and testing sets. We normalize the input data to ensure that all values are between 0 and 1. Finally, we train the model for 100 epochs and validate the results using the testing set.
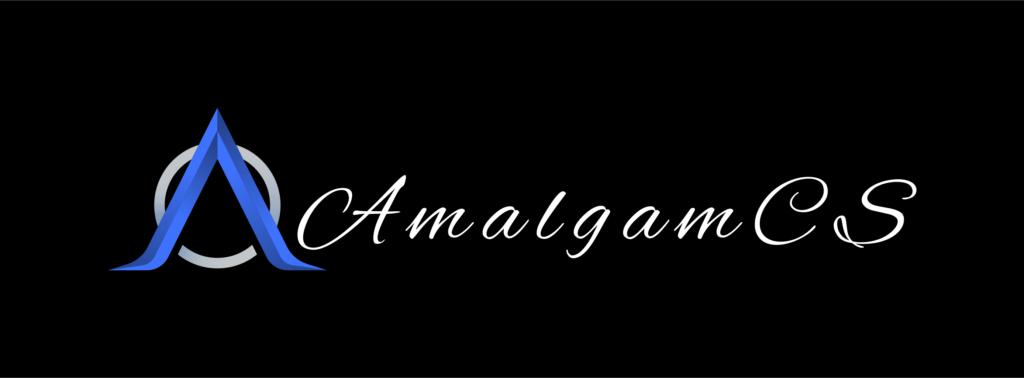